How Developers Can Enhance Their Debugging Competencies By Gustavo Woltmann
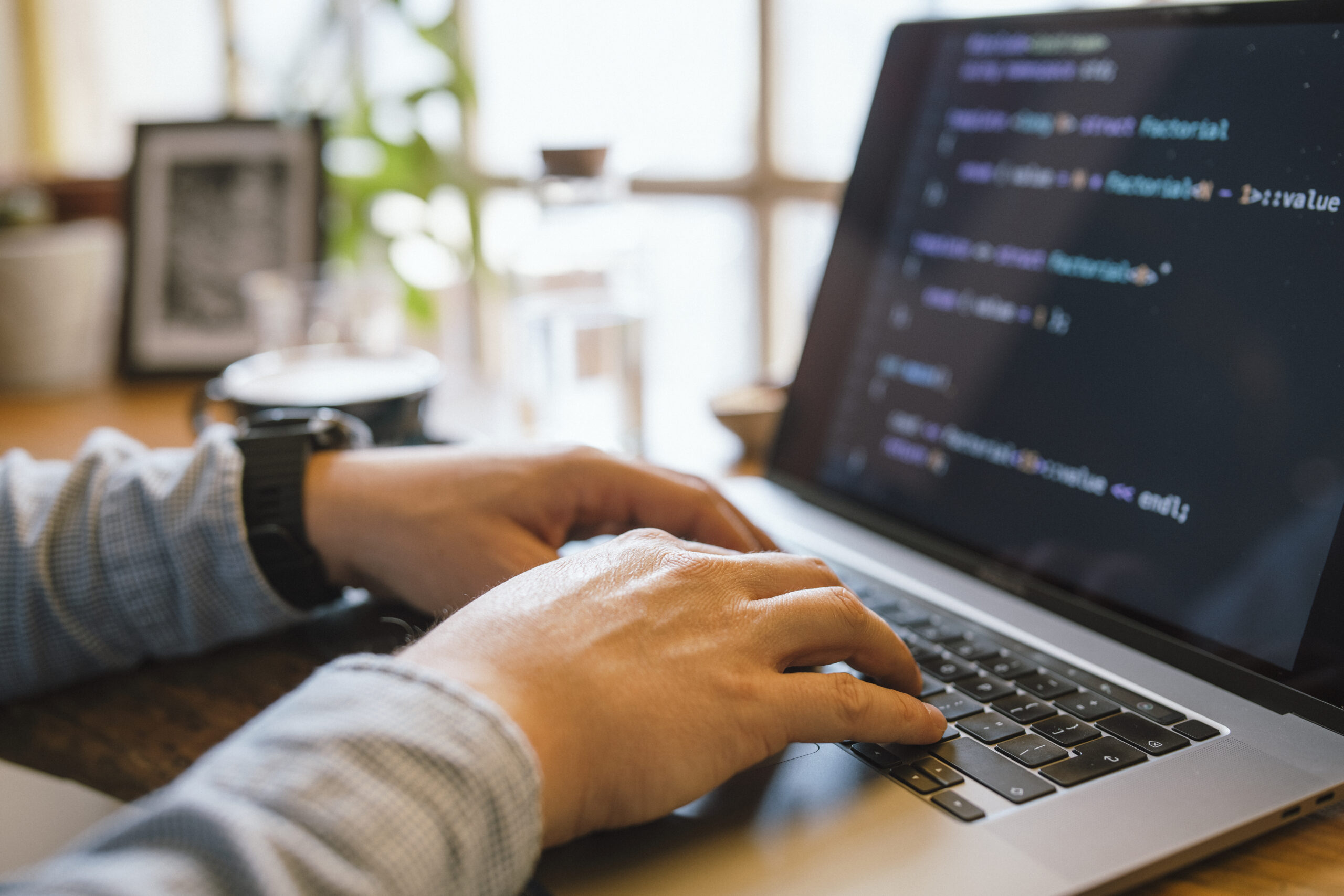
Debugging is Just about the most critical — but typically neglected — competencies inside of a developer’s toolkit. It's not just about fixing broken code; it’s about being familiar with how and why things go wrong, and Studying to Believe methodically to solve issues successfully. Irrespective of whether you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hours of aggravation and significantly enhance your productivity. Here are several procedures to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
Among the quickest methods developers can elevate their debugging competencies is by mastering the instruments they use every single day. Although composing code is a single part of enhancement, figuring out the way to interact with it properly in the course of execution is equally vital. Modern-day growth environments come Geared up with potent debugging abilities — but a lot of developers only scratch the area of what these instruments can do.
Get, as an example, an Integrated Progress Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications allow you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When used correctly, they Enable you to observe just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, observe community requests, perspective actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can convert frustrating UI troubles into workable tasks.
For backend or program-stage developers, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Regulate over working procedures and memory administration. Understanding these instruments may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into comfy with Edition Management devices like Git to understand code background, uncover the precise moment bugs had been launched, and isolate problematic improvements.
Finally, mastering your tools signifies likely beyond default settings and shortcuts — it’s about building an intimate understanding of your growth natural environment in order that when concerns come up, you’re not dropped at nighttime. The higher you recognize your instruments, the greater time you could expend resolving the particular dilemma as an alternative to fumbling by way of the procedure.
Reproduce the condition
One of the more important — and sometimes neglected — methods in powerful debugging is reproducing the situation. In advance of jumping in to the code or making guesses, builders need to have to make a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a game of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is accumulating as much context as possible. Check with inquiries like: What actions triggered The problem? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to isolate the exact ailments below which the bug takes place.
When you’ve gathered adequate info, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting a similar info, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about composing automated checks that replicate the edge situations or point out transitions involved. These exams don't just assist expose the problem but additionally protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it would transpire only on sure operating techniques, browsers, or underneath particular configurations. Utilizing applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a action — it’s a state of mind. It needs endurance, observation, and also a methodical tactic. But as you can consistently recreate the bug, you're previously midway to repairing it. That has a reproducible state of affairs, You may use your debugging applications more effectively, examination likely fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an summary criticism right into a concrete problem — and that’s the place developers thrive.
Browse and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. Instead of viewing them as aggravating interruptions, developers should really master to deal with error messages as immediate communications with the procedure. They generally inform you just what happened, where by it took place, and at times even why it happened — if you know how to interpret them.
Get started by looking at the concept carefully As well as in total. Numerous builders, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But further during the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines — read through and recognize them initial.
Split the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Does it position to a specific file and line variety? What module or function activated it? These questions can tutorial your investigation and stage you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and Studying to acknowledge these can greatly quicken your debugging course of action.
Some errors are vague or generic, and in Those people circumstances, it’s very important to examine the context during which the mistake happened. Check associated log entries, input values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings possibly. These frequently precede more substantial difficulties and provide hints about possible bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a additional economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised proficiently, it provides real-time insights into how an software behaves, helping you understand what’s happening underneath the hood without having to pause execution or move in the code line by line.
A great logging technique starts with knowing what to log and at what level. Common logging degrees include things like DEBUG, Details, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info during development, Facts for normal functions (like thriving start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Lethal if the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and slow down your method. Concentrate on key gatherings, state variations, enter/output values, and demanding conclusion factors inside your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially important in manufacturing environments where by stepping by means of code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could reduce the time it will require to identify problems, get deeper visibility into your programs, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a technical undertaking—it is a form of investigation. To efficiently discover and take care of bugs, developers should strategy the method just like a detective solving a mystery. This attitude will help stop working advanced challenges into manageable parts and stick to clues logically to uncover the basis induce.
Start by gathering evidence. Look at the symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys against the law scene, obtain just as much applicable information and facts as you could without leaping to conclusions. Use logs, exam conditions, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request your self: What might be creating this conduct? Have any modifications recently been made into the codebase? Has this challenge transpired just before under similar situation? The purpose will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the trouble in a managed surroundings. In case you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you closer to the reality.
Pay out shut consideration to little aspects. Bugs usually hide while in the least predicted areas—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface afterwards.
Finally, retain notes on Everything you tried out and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for long run problems and support Many others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, solution issues methodically, and turn into more practical at uncovering concealed issues in sophisticated programs.
Generate Tests
Creating exams is among the most effective methods to increase your debugging techniques and In general development efficiency. Tests not just support catch bugs early but in addition function a security net that gives you confidence when creating adjustments to the codebase. A very well-analyzed software is simpler to debug as it allows you to pinpoint exactly exactly where and when a problem occurs.
Get started with device assessments, which center on particular person features or modules. These modest, isolated assessments can promptly expose no matter whether a certain piece of logic is Operating as expected. When a exam fails, you straight away know wherever to glance, drastically lowering enough time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following Beforehand staying mounted.
Up coming, combine integration tests and conclusion-to-conclude tests into your workflow. These help be sure that a variety of areas of your software get the job done collectively smoothly. They’re specially beneficial for catching bugs that take place in complex units with several components or expert services interacting. If one thing breaks, your checks can inform you which part of the pipeline unsuccessful and below what ailments.
Creating exams also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, envisioned outputs, and edge situations. This volume of comprehension naturally potential customers to better code framework and fewer bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you may concentrate on correcting the bug and view your examination go when the issue is settled. This technique ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the aggravating guessing match right into a structured and predictable process—assisting you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—observing your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is solely stepping absent. Getting breaks can help you reset your intellect, cut down frustration, and sometimes see the issue from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. During this point out, your Mind gets much less efficient at problem-resolving. A brief stroll, a coffee crack, or simply switching to a unique process for ten–quarter-hour can refresh your target. Several developers report getting the basis of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate within the background.
Breaks also assistance avert burnout, Primarily for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that time to maneuver about, extend, or do something unrelated to code. It may well really feel counterintuitive, Primarily below limited deadlines, however it essentially leads to more rapidly and more effective debugging Over time.
To put it briefly, using breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is a component of resolving it.
Learn From Every single Bug
Each individual bug you encounter is much more than simply A short lived setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you anything precious for those who make an effort to mirror and examine what went Mistaken.
Start out by inquiring you a few important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught previously with superior practices like unit tests, code reviews, or logging? The responses often reveal blind places in the workflow or understanding and help you build much better coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll begin to see designs—recurring problems get more info or typical mistakes—you could proactively prevent.
In crew environments, sharing Whatever you've discovered from the bug with the peers could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as essential portions of your improvement journey. In fact, several of the best developers are not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you fix adds a completely new layer in your ability established. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills will take time, exercise, and patience — nevertheless the payoff is big. It will make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.